In the Appendix of this Tutorial, I will introduce useful Android common library/function. These are usually applicable not only for Android TV, but also for Android phone/tablet device as well.
Spinner Fragment – show loading progress bar
While user is downloading big contents from web, you might want to show progress bar to notify user that some process (in this time downloading) is being done in background. It helps navigating user to wait this process.
Here, SpinnerFragment can be used to show circle arrow progress bar. Starting by making class by right click on “ui” package → [New] → [Java Class] → put Name as “SpinnerFragment”.
This class is a subclass of Fragment, and it is just making ProgressBar in onCreateView
package com.corochann.androidtvapptutorial.ui; import android.app.Fragment; import android.os.Bundle; import android.view.Gravity; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.FrameLayout; import android.widget.ProgressBar; /** * SpinnerFragment shows spinning progressbar to notify user that * application is processing something (while downloading, or preparing sth etc.) * * Example of usage in AsyncTask * + Start showing: OnPreExecute * mSpinnerFragment = new SpinnerFragment(); * getFragmentManager().beginTransaction().add(R.id.some_view_group, mSpinnerFragment).commit(); * + Stop showing: OnPostExecute * getFragmentManager().beginTransaction().remove(mSpinnerFragment).commit(); */ public class SpinnerFragment extends Fragment { private static final String TAG = SpinnerFragment.class.getSimpleName(); private static final int SPINNER_WIDTH = 100; private static final int SPINNER_HEIGHT = 100; @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { ProgressBar progressBar = new ProgressBar(container.getContext()); if (container instanceof FrameLayout) { FrameLayout.LayoutParams layoutParams = new FrameLayout.LayoutParams(SPINNER_WIDTH, SPINNER_HEIGHT, Gravity.CENTER); progressBar.setLayoutParams(layoutParams); } return progressBar; } }
Usage of Spinner Fragment – AsyncTask
Put button in MainFragment
To introduce the usage of SpinnerFragment, make button to show this Fragment. I will make “Spinner” button in MainFragment.
public class MainFragment extends BrowseFragment { ... private static final String GRID_STRING_SPINNER = "Spinner"; ... private void loadRows() { mRowsAdapter = new ArrayObjectAdapter(new ListRowPresenter()); /* GridItemPresenter */ HeaderItem gridItemPresenterHeader = new HeaderItem(0, "GridItemPresenter"); GridItemPresenter mGridPresenter = new GridItemPresenter(); ArrayObjectAdapter gridRowAdapter = new ArrayObjectAdapter(mGridPresenter); gridRowAdapter.add(GRID_STRING_ERROR_FRAGMENT); gridRowAdapter.add(GRID_STRING_GUIDED_STEP_FRAGMENT); gridRowAdapter.add(GRID_STRING_RECOMMENDATION); gridRowAdapter.add(GRID_STRING_SPINNER); mRowsAdapter.add(new ListRow(gridItemPresenterHeader, gridRowAdapter)); ... /* Set */ setAdapter(mRowsAdapter); } ... private final class ItemViewClickedListener implements OnItemViewClickedListener { @Override public void onItemClicked(Presenter.ViewHolder itemViewHolder, Object item, RowPresenter.ViewHolder rowViewHolder, Row row) { ... } else if (item == GRID_STRING_SPINNER) { // Show SpinnerFragment, while doing some is executed. new ShowSpinnerTask().execute(); } } } }
After click “Spinner” button, ShowSpinnerTask starts, which is explained following.
AsyncTask implementation
Use case of SpinnerFragment is when something is processed in background, and AsyncTask is a one popular choice to do something in background.
Below, ShowSpinnerTask is introduced as a innerclass of MainFragment. It is mock example how to use SpinnerFragment. SpinnerFragment is added to the MainFragment before background process starts (onPreExecute), and removed after background process finishes (onPostExecute). This add and remove is done by using FragmentManager.
Adding and removing SpinnerFragment is enough. We don’t need to specify any animation, and UI update process during the background process is required.
In the first argument of add method, we need to specify an layout id of ViewGroup to attach SpinnerFragment. In this example main_browse_fragment is used.
private class ShowSpinnerTask extends AsyncTask<Void, Void, Void> { SpinnerFragment mSpinnerFragment; @Override protected void onPreExecute() { mSpinnerFragment = new SpinnerFragment(); getFragmentManager().beginTransaction().add(R.id.main_browse_fragment, mSpinnerFragment).commit(); } @Override protected Void doInBackground(Void... params) { // Do some background process here. // It just waits 5 sec in this Tutorial SystemClock.sleep(5000); return null; } @Override protected void onPostExecute(Void aVoid) { getFragmentManager().beginTransaction().remove(mSpinnerFragment).commit(); } }
Build and run
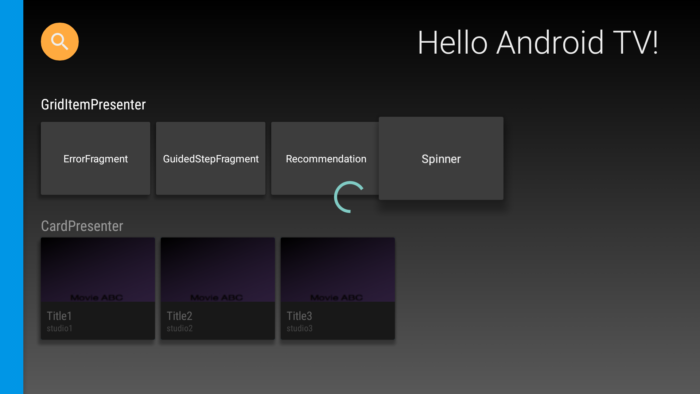
When “Spinner” button is pressed, circle type progress bar will appear.
Source code is on github.